FULL STACK JAVA DEVELOPMENT
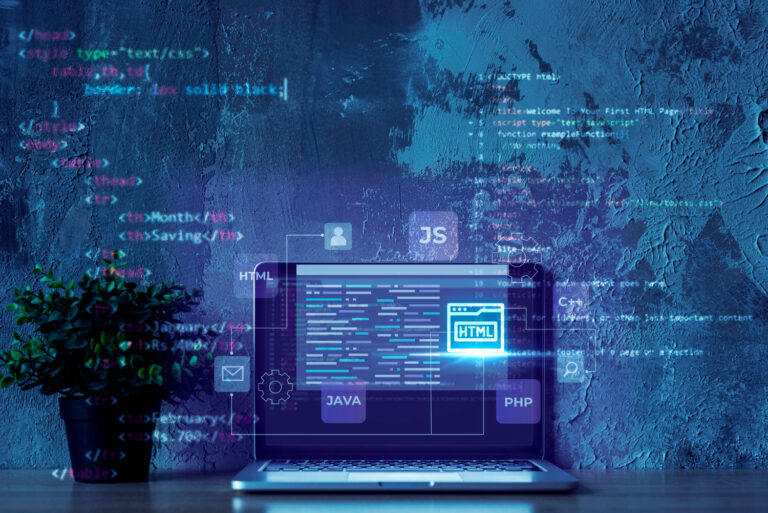
100% Job Guaranteed Program*
The Full Stack Java Development Course will help you start your career as a Java Developer and become a ICMT certified web developer. It involves developing both the front-end and back-end components of a web application using Java technologies. It requires proficiency in Java programming, as well as knowledge of frameworks and tools for building user interfaces, handling server-side logic, and managing databases. A Full Stack Java developer can create responsive user interfaces using HTML, CSS, and JavaScript, implement server-side functionality using Java frameworks like Spring, and integrate databases using technologies such as JDBC. This comprehensive approach enables developers to design and build end-to-end web applications that deliver seamless user experiences and efficient data processing.
Module 1 – Java
· Introduction and Software Installation
· Basic of Java
· Data Types
· Operators in Java
· Control Statement and Looping
· Arrays and Strings
· Collection interfaces (List, Set, Map)
· Functions
· OOP’s in Python
· File Handling
· Exception Handling
· Multi-Threading
· Hands-on Sessions and Assignments for Practice
· Java – Graded Exam
Module 2 – Data Structures and Algorithms
· Arrays
· linked lists
· Stacks
· Queues
· Trees
· Graphs
· Hash Tables
· Searching Algorithms
· Sorting Algorithms
Module 3 – Database Connectivity
- Introduction to databases and SQL
- Java Database Connectivity (JDBC) API
- CRUD operations using JDBC
- Connection Pooling
- Performance Optimization
Module 4 – Web Development Basics
· Introduction and Software Installation
· Introduction to web development and HTTP
· HTML, CSS, and JavaScript fundamentals
· DOM manipulation and event handling
· Introduction to front-end frameworks (e.g., React, Angular, Vue.js)
Module 5 – Servlets & JSP
· Introduction to servlets and JavaServer Pages (JSP)
· Servlet lifecycle and request/response handling
· JSP scripting elements, and JSP standard tag library (JSTL)
· Session management and cookies
Module 6 – Spring Framework
· Introduction to the Spring Framework
· Dependency injection and inversion of control (IoC)
· Spring MVC for building web applications
· Data access with Spring JDBC and ORM frameworks (e.g., Hibernate)
Module 7 – RESTful Webservices
- Introduction to RESTful Architecture
· Building RESTful APIs with Java and Spring
· JSON and XML data serialization
· Authentication and authorization in RESTful services
Module 8 – Front – end Development
- Advanced JavaScript concepts (closures, prototypes)
- Front-end build tools (Webpack, Babel)
- Styling with CSS preprocessors (Sass, Less)
- Single-page application (SPA) development using a front-end framework
Module 9 – Security and Authentication
· Web application security principles
· Cross-Site Scripting (XSS) and Cross-Site Request Forgery (CSRF)
· User authentication and authorization
· Role-based access control (RBAC)
Module 10 – Testing and Quality Assurance
· Unit testing with JUnit and Mockito
· Integration testing and end-to-end testing
· Code coverage and quality metrics
· Test-driven development (TDD) principles
Module 11 – Deployment and DevOps
· Deployment strategies (on-premises, cloud-based)
· Continuous Integration and Continuous Deployment (CI/CD)
· Docker containers and containerization
· Monitoring and logging in production environments
Module 12 – Advanced Java Topics
· Java 8 features (lambda expressions, streams)
· Functional programming in Java
· Reactive programming with Java and Spring WebFlux
· Performance tuning and optimization techniques